Hi there,
Here I am again with the second part of the Jinja course. Last time we talked about expressions, statements, comments, variables, and filters. Today we’ll continue with tests, multiline comments, whitespace control, escaping, and multiline filters. Let’s get started quickly!
โญโญโญ NOTE: โญโญโญ
This article accompanies a YouTube video. I wrote it for people who would rather read than watch a video. To keep doing this, I would like to ask you to check out the video, leave a comment under the video, give the video a thumbs up, and subscribe to my YouTube channel. This means that the video is offered more often to new visitors so that they also stay informed of the latest Home Assistant tutorials.
Thank you for your support!
Ed
Tests
Last time we ended with filters, which allow you to convert a value into another output. If you haven’t watched the previous episode yet, start by viewing the playlist. You can find the link in the description of this video. In addition to filters, you can also use “Tests”. Tests can be used to check if a variable or a value meets certain requirements.
Let’s revisit our example from last time. Remember, we looked at the status of our home zone. It was 1, but because a value is always returned as a string, we couldn’t simply check if the content contained the number 1. If we had known in advance that the value was returned as a string, we could have immediately converted it to an integer.
This way, we could pre-check if the value of a sensor is a number:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{% if presencestatus is number() %}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
You see that the presence status is 1 and seems to be a number, but when you test if the presence status is a number, it turns out not to be the case. If you then test if the presence status is a string, you’ll see that the test is successful.
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{% if presencestatus is string() %}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
For example, you can quickly check if a value is even or odd by converting the value to an integer and then testing if it is odd:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{% if presencestatus | int is odd() %}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
First, the presence status is converted to an integer using a filter, and then you test if it is odd. You can see that the test is successful now.
The list of built-in tests can be found on this page. You can find the link in the video description.
Multi-line comments
In episode 1, I showed you how to add comments to your code. However, sometimes it’s also useful to enclose a piece of code within comment tags so that you don’t have to delete the code and can reuse it later. For that, you place the entire piece of code between braces and hashtags like in this example:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{#
{% if presencestatus | int is odd() %}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
#}
That entire piece of code won’t be used anymore, but you can easily reuse it later by removing the braces and hashtags again.
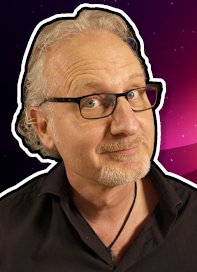
I need your help!
You will be doing me a huge favor if you subscribe to my channel if you haven’t already. And, you will help me a lot if you also give this video a thumbs up and leave a comment. This way, YouTube will present this video to new people, making the channel grow! In the video description, you will also find information about how you can sponsor me so that I can continue to make these tutorials for you.
Thank you!
Whitespace Control
In the example I just showed, you could see that there was a blank line between the two lines “The presence status is: 1” and “The result of the test is: Correct”. Additionally, the second line was indented with a few spaces. To prevent that, you have a few options that you can apply in your statement rules.
The blank line is displayed because the statement rules are also seen as separate lines. Consequently, they are displayed as blank lines. This can be annoying if, for example, you want to display text in a custom sensor or a card on your dashboard. If you want to prevent the blank line from being displayed, you place a minus sign after the opening brace and the percent sign of the statement:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{%- if presencestatus | int is odd() %}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
You can see that the blank line is now gone. However, the line is still indented! If you want the blank line not to be displayed and the line not to be indented, you place a minus sign before the closing brace and the percent sign that precedes the line you want to correct:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{% if presencestatus | int is odd() -%}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
Now you can see that the blank line has disappeared and the text is no longer indented.
You can also place a minus sign on both sides of the statement. In that case, all blank lines and leading spaces are removed:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{%- if presencestatus | int is odd) -%}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
Now you can see that two lines are stuck together.
Escaping
Occasionally, it’s preferableโperhaps even essentialโfor Jinja to disregard sections that it would typically interpret as variables or blocks. For instance, if you wish to treat double curly braces as a literal string within a template without triggering variable processing using the default syntax, you’ll need to employ a workaround.
The simplest way to do this is by putting single quotes around the string you want to use. If I don’t use them, I’ll get an error message:
{{ {{ }}
However, if I do put single quotes around the double braces, you’ll see that the double braces are interpreted as a string:
{{ '{{' }}
This way, you can also use more extensive strings in combination with values, as you can see in this example:
{{ 'The presencestatus is: ' + states('zone.home') }}
You see that here I start with the string “The presence status is: ” and append to it the value of zone.home.
If you want to use entire blocks of text now, you can mark them with the raw tag as you see in this example:
{% set presencestatus = states('zone.home') %}
The presencestatus is: {{ presencestatus }}
{% raw %}
{%- if presencestatus | int is even() -%}
The result of the test is: Correct
{% else %}
The result of the test is: Not Correct
{% endif %}
{% endraw %}
As you can see, the code is now printed on the screen not as code, but as a string. I’ve never used this option, but perhaps you can find some use for it.
Multiline Filters
In the previous episode, I talked about filters and how you can apply them to values using a pipe symbol. But you can also apply filters to multiple lines. For that, you use the filter tag. For example, see this instance:
{% filter upper %}
This text becomes uppercase
And this text too.
{% endfilter %}
As you can see, all text between the filter tags is displayed in uppercase. See this page for the complete list of filters that you can use.
So, this was episode 2 of the Jinja course. You now have the basic components under your belt. In the next episode, I’ll explain how control structures like For, If-Then, and Macros work in Jinja and Home Assistant.
Thank you for watching, and if my work helps you, please consider sponsoring me monthly, just like these amazing people do. This channel is my job, and unfortunately, the income via YouTube is too little to live on. Without your help, I can’t continue this channel. You’ll find the links to Patreon, Ko-Fi, and how to become a channel member in the video description. Thank you!
And, don’t forget to give this video a thumbs up, subscribe to my channel, and leave a comment below this video. That helps to grow this channel!
I’ll see you soon in my next video.
Bye Bye!